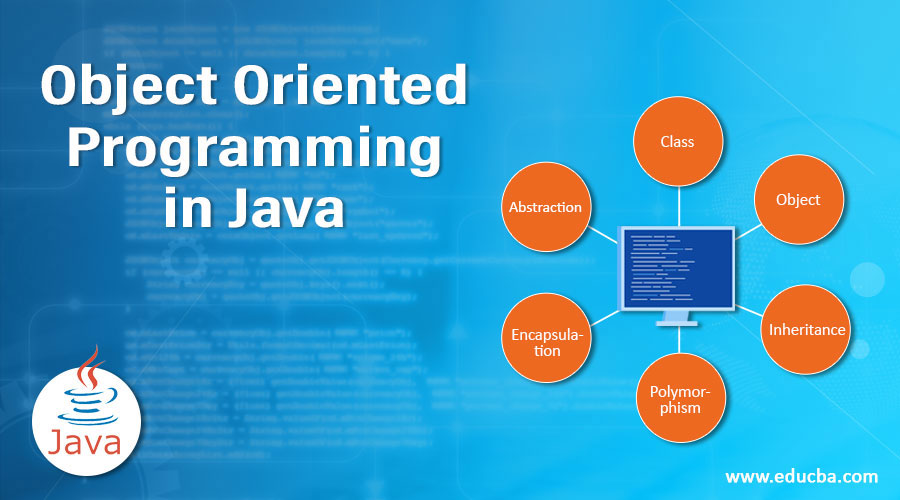
This course explores advanced concepts of object-oriented programming (OOP) using Java, emphasizing design patterns, principles, and best practices for building robust and maintainable software applications.
Key topics include:
-Advanced OOP Concepts: In-depth study of inheritance, polymorphism, encapsulation, and abstraction.
-Design Patterns: Introduction to common design patterns (e.g., Singleton, Factory, Observer) and their practical applications in software development.
-Exception Handling: Advanced techniques for managing exceptions, creating custom exceptions, and ensuring code robustness.
-Generics and Collections: Using generics for type safety and exploring Java Collections Framework for efficient data management.
-Functional Programming in Java: Introduction to functional programming concepts, including lambdas, streams, and higher-order functions.
-Multithreading and Concurrency: Understanding threads, synchronization, and concurrent data structures for building high-performance applications.
-Software Design Principles: Applying SOLID principles and other best practices for scalable and maintainable code.
By the end of this course, students will have a deep understanding of advanced OOP concepts and the ability to apply these principles effectively in their Java programming projects.
Prerequisites: Intermediate knowledge of Java and OOP principles.
- Teacher: Kingsley Drah
- Teacher: Dr. Dominic Asamoah